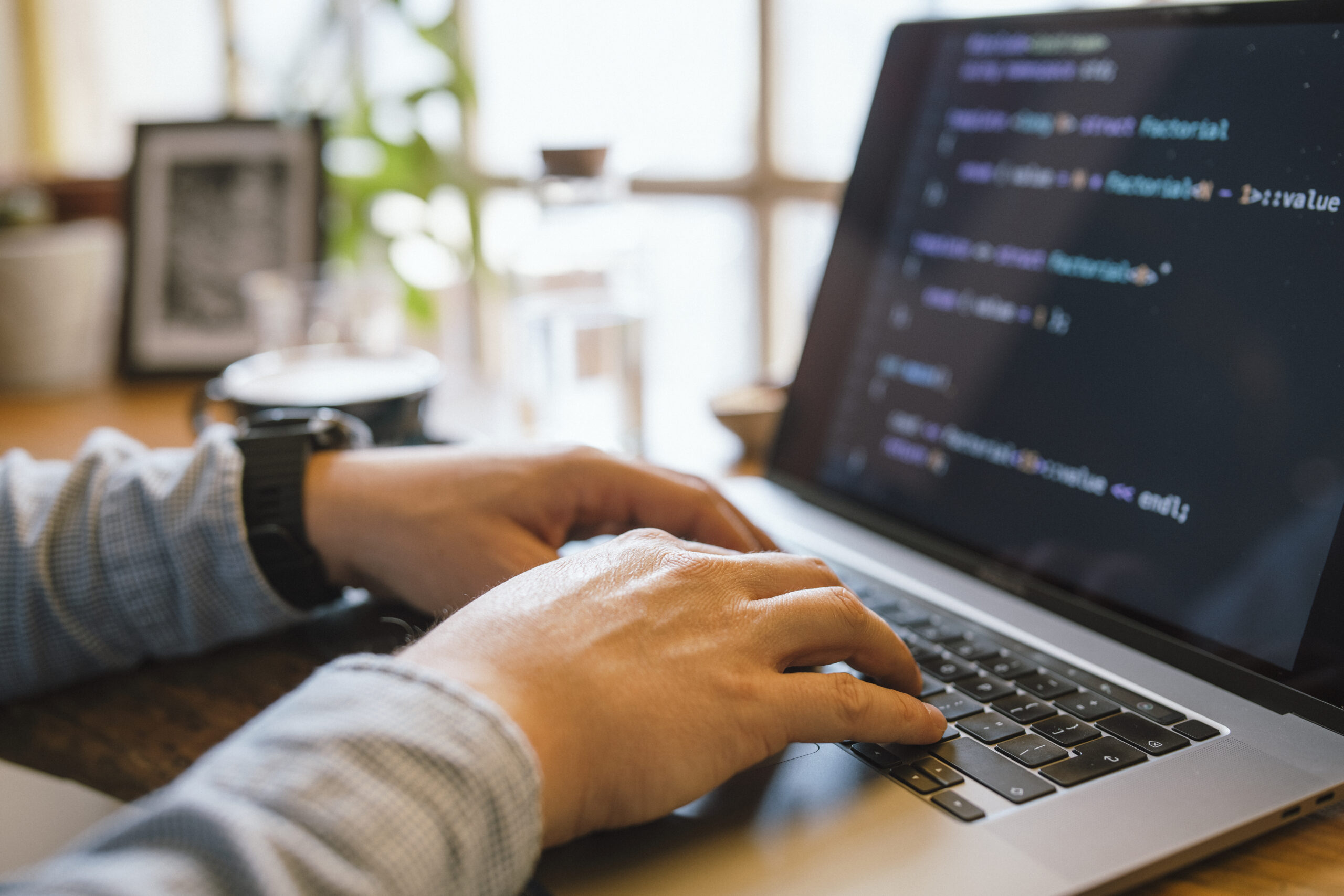
Debugging is Just about the most crucial — but generally neglected — competencies in a developer’s toolkit. It's not pretty much correcting broken code; it’s about knowing how and why factors go Erroneous, and Studying to Assume methodically to solve issues successfully. Whether you're a newbie or even a seasoned developer, sharpening your debugging capabilities can preserve hrs of annoyance and radically help your productivity. Here are several methods to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest approaches developers can elevate their debugging expertise is by mastering the resources they use daily. Whilst creating code is 1 A part of development, knowing ways to connect with it proficiently for the duration of execution is equally essential. Modern progress environments arrive equipped with powerful debugging abilities — but several developers only scratch the floor of what these resources can perform.
Just take, as an example, an Integrated Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and perhaps modify code within the fly. When utilized correctly, they Enable you to observe particularly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-end builders. They allow you to inspect the DOM, keep an eye on community requests, check out serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can flip disheartening UI concerns into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of functioning procedures and memory administration. Studying these instruments can have a steeper Studying curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Manage programs like Git to be familiar with code history, uncover the precise instant bugs were being introduced, and isolate problematic improvements.
Ultimately, mastering your tools implies heading further than default settings and shortcuts — it’s about creating an intimate understanding of your growth ecosystem so that when problems come up, you’re not misplaced at nighttime. The higher you understand your equipment, the more time it is possible to commit fixing the actual difficulty rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more significant — and infrequently forgotten — techniques in productive debugging is reproducing the situation. In advance of leaping to the code or producing guesses, developers require to create a consistent environment or scenario where by the bug reliably seems. Devoid of reproducibility, repairing a bug turns into a recreation of opportunity, frequently leading to squandered time and fragile code alterations.
Step one in reproducing an issue is gathering just as much context as is possible. Talk to issues like: What actions resulted in the issue? Which ecosystem was it in — progress, staging, or generation? Are there any logs, screenshots, or error messages? The more element you might have, the less complicated it gets to isolate the exact conditions under which the bug takes place.
As soon as you’ve collected sufficient information and facts, try and recreate the issue in your neighborhood atmosphere. This may indicate inputting the same facts, simulating comparable person interactions, or mimicking program states. If The difficulty appears intermittently, look at creating automatic tests that replicate the edge conditions or state transitions associated. These tests not merely assistance expose the trouble but will also avoid regressions Sooner or later.
Occasionally, The problem may very well be surroundings-precise — it'd occur only on selected operating techniques, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It requires persistence, observation, plus a methodical solution. But once you can regularly recreate the bug, you are presently midway to repairing it. That has a reproducible state of affairs, You can utilize your debugging equipment additional effectively, test prospective fixes safely and securely, and converse far more Evidently with your team or users. It turns an abstract complaint right into a concrete problem — and that’s in which developers thrive.
Go through and Fully grasp the Mistake Messages
Error messages are frequently the most useful clues a developer has when anything goes Improper. As opposed to viewing them as irritating interruptions, developers should learn to treat mistake messages as immediate communications through the technique. They usually let you know just what occurred, exactly where it transpired, and in some cases even why it took place — if you know how to interpret them.
Begin by examining the concept very carefully and in whole. A lot of developers, specially when beneath time pressure, look at the primary line and quickly commence making assumptions. But further while in the error stack or logs may lie the genuine root lead to. Don’t just copy and paste mistake messages into serps — read through and comprehend them first.
Split the error down into sections. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it issue to a particular file and line number? What module or purpose triggered it? These issues can manual your investigation and place you toward the liable code.
It’s also useful to be familiar with the terminology in the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and Understanding to acknowledge these can considerably quicken your debugging course of action.
Some errors are obscure or generic, As well as in These situations, it’s crucial to examine the context wherein the mistake happened. Examine similar log entries, input values, and recent variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages are usually not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues more quickly, lessen debugging time, and turn into a extra efficient and assured developer.
Use Logging Properly
Logging is The most powerful resources within a developer’s debugging toolkit. When applied effectively, it provides true-time insights into how an software behaves, aiding you recognize what’s occurring beneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information during development, INFO for typical situations (like prosperous start out-ups), WARN for prospective problems that don’t break the applying, Mistake for true difficulties, and Deadly when the system can’t continue on.
Steer clear of flooding your logs with excessive or irrelevant data. An excessive amount of logging can obscure important messages and decelerate your technique. Give attention to important events, condition adjustments, input/output values, and significant selection points in the code.
Structure your log messages Evidently and continually. Include context, such as timestamps, ask for IDs, and function names, so it’s much easier to trace issues in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what circumstances are achieved, and what branches of logic are executed—all without having halting the program. They’re Primarily useful in production environments the place stepping via code isn’t doable.
In addition, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. With a properly-thought-out logging technique, you are able to decrease the time it's going to take to spot troubles, gain deeper visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To efficiently establish and take care of bugs, developers have to approach the process just like a detective fixing a secret. This mindset aids break down advanced challenges into manageable components and comply with clues logically to uncover the basis induce.
Begin by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or general performance problems. Much like a detective surveys a criminal offense scene, gather as much appropriate details as it is possible to with no leaping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear picture of what’s happening.
Next, type hypotheses. Request on your own: What may very well be resulting in this habits? Have any alterations just lately been created towards the codebase? Has this problem occurred right before less than related conditions? The aim would be to slender down alternatives and establish probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue in a managed setting. In case you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, request your code concerns and Enable the final results lead you nearer to the truth.
Spend shut consideration to small facts. Bugs usually hide during the minimum expected spots—like a lacking semicolon, an off-by-a single mistake, or maybe a race situation. Be complete and individual, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may perhaps conceal the actual difficulty, just for it to resurface later.
And lastly, maintain notes on That which you tried and figured out. Just as detectives log their investigations, documenting your debugging approach can save time for foreseeable future issues and support others realize your reasoning.
By wondering like a detective, builders can sharpen their analytical skills, strategy complications methodically, and come to be more effective at uncovering concealed issues in intricate programs.
Create Assessments
Writing exams is among the simplest approaches to transform your debugging capabilities and Over-all improvement effectiveness. Checks don't just assist catch bugs early and also function a security Web that gives you self-confidence when producing variations for your codebase. A effectively-analyzed application is easier to debug since it permits you to pinpoint just wherever and when a challenge takes place.
Get started with device checks, which deal with individual capabilities or Developers blog modules. These small, isolated tests can quickly expose regardless of whether a particular piece of logic is Operating as predicted. Each time a check fails, you right away know exactly where to appear, considerably decreasing the time spent debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear immediately after Earlier currently being mounted.
Following, integrate integration tests and end-to-end checks into your workflow. These enable be sure that several areas of your application work alongside one another efficiently. They’re notably beneficial for catching bugs that occur in advanced programs with numerous factors or companies interacting. If one thing breaks, your checks can inform you which A part of the pipeline unsuccessful and below what conditions.
Producing tests also forces you to definitely Consider critically about your code. To check a attribute correctly, you would like to grasp its inputs, expected outputs, and edge scenarios. This degree of being familiar with Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, composing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check go when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In brief, composing checks turns debugging from the irritating guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s quick to become immersed in the issue—watching your display screen for hrs, hoping Alternative right after Resolution. But One of the more underrated debugging applications is simply stepping absent. Taking breaks helps you reset your thoughts, decrease stress, and sometimes see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could possibly start off overlooking evident glitches or misreading code that you just wrote just hrs previously. On this state, your brain results in being fewer economical at trouble-resolving. A short walk, a coffee break, or simply switching to another endeavor for ten–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job in the history.
Breaks also support avoid burnout, Particularly during for a longer period debugging periods. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electrical power plus a clearer attitude. You might quickly recognize a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in speedier and more effective debugging In the long term.
In short, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is usually a mental puzzle, and rest is an element of solving it.
Find out From Just about every Bug
Each and every bug you face is a lot more than just a temporary setback—It can be a possibility to develop like a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, every one can instruct you anything precious if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots within your workflow or knowing and allow you to Create more powerful coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After some time, you’ll begin to see patterns—recurring problems or common mistakes—you could proactively prevent.
In staff environments, sharing Whatever you've discovered from the bug with all your friends may be especially impressive. No matter whether it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, supporting Other people steer clear of the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. Instead of dreading bugs, you’ll start off appreciating them as crucial parts of your growth journey. In any case, a lot of the ideal developers will not be the ones who publish ideal code, but individuals that constantly master from their blunders.
Eventually, Every single bug you take care of adds a different layer for your ability established. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and persistence — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.